Upload file to Firebase Storage as a Formdata using Multer
Many times we need to upload files to some storage system using multipart/form-data as an HTTP request body. And that could be the best way to upload files to any Cloud storage or server. Let's see how we can do that using NodeJS and Firebase storage.
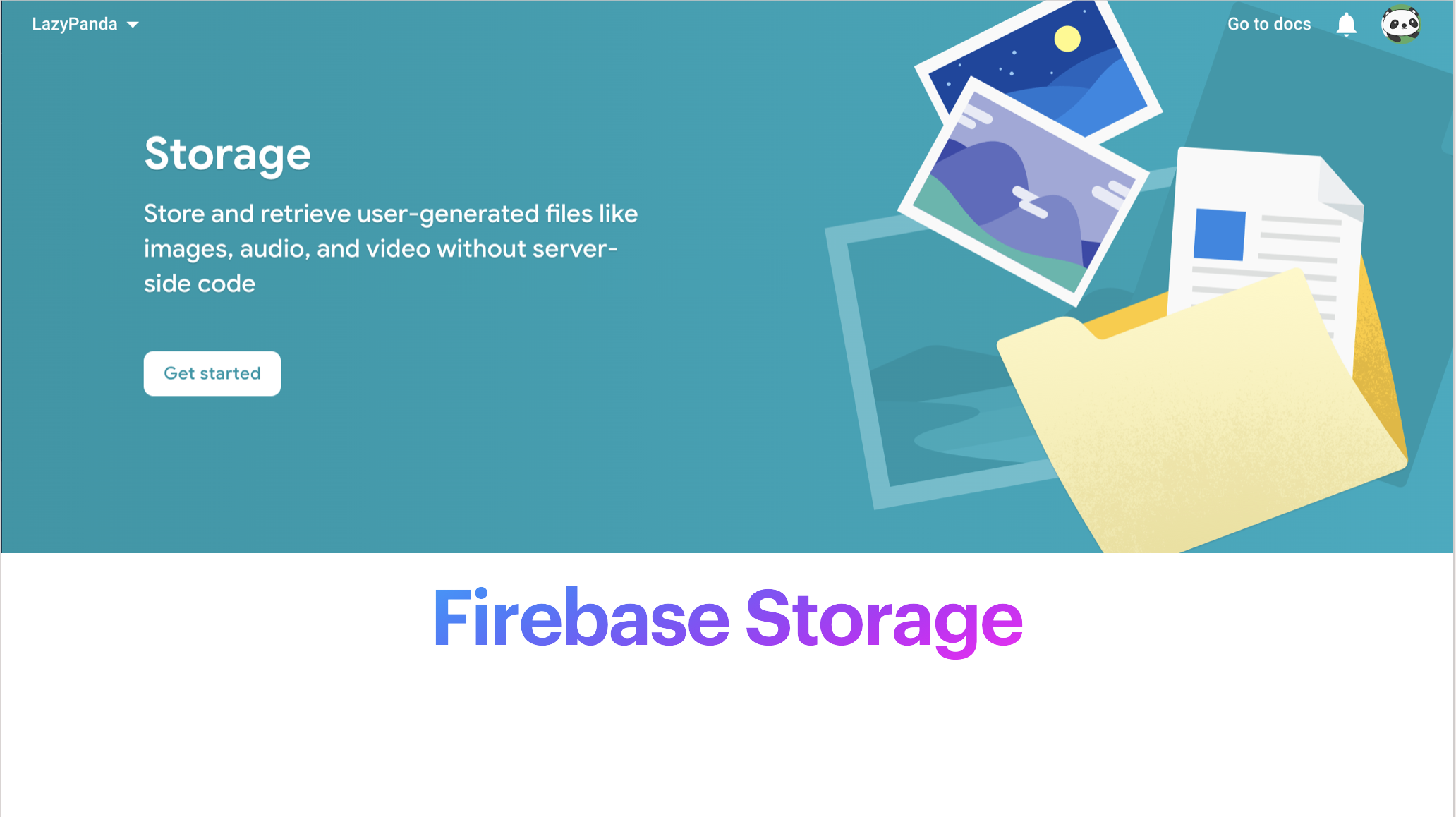
Step 1: Firebase Account setup and enabled Firebase Storage.
To do that please follow the link 'https://firebase.google.com/docs/storage/web/start' and get started.
Step 2: Make the Firebase storage publicly accessible.
Based on application needs, if the storage is behind the login screen then there is no need to make the storage publicly accessible read / write. But in my case, I am uploading files without login to google cloud. I have to make the storage publicly accessible. The rule is being set as below -
rules_version = '2';
service firebase.storage {
match /b/{bucket}/o {
match /{allPaths=**} {
allow read, write;
}
}
}
Step 3: Save and keep google config (help to connect Firebase from NodeJS)
Firebase Project config
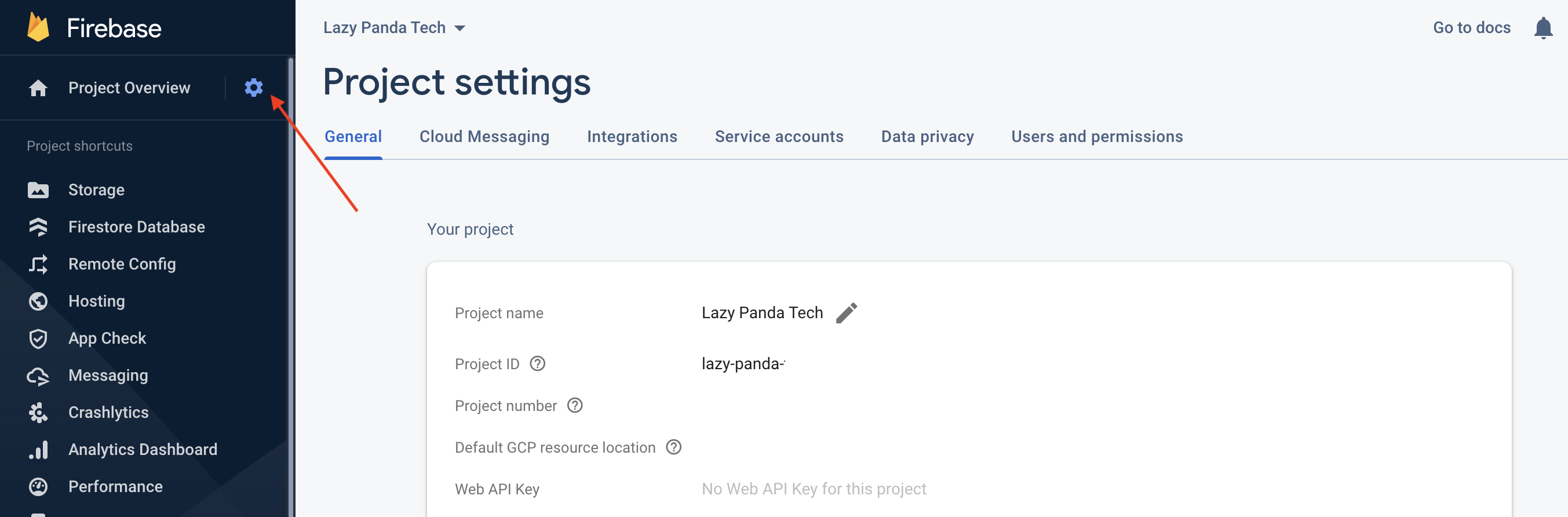
Firebase Config will be used in NodeJS
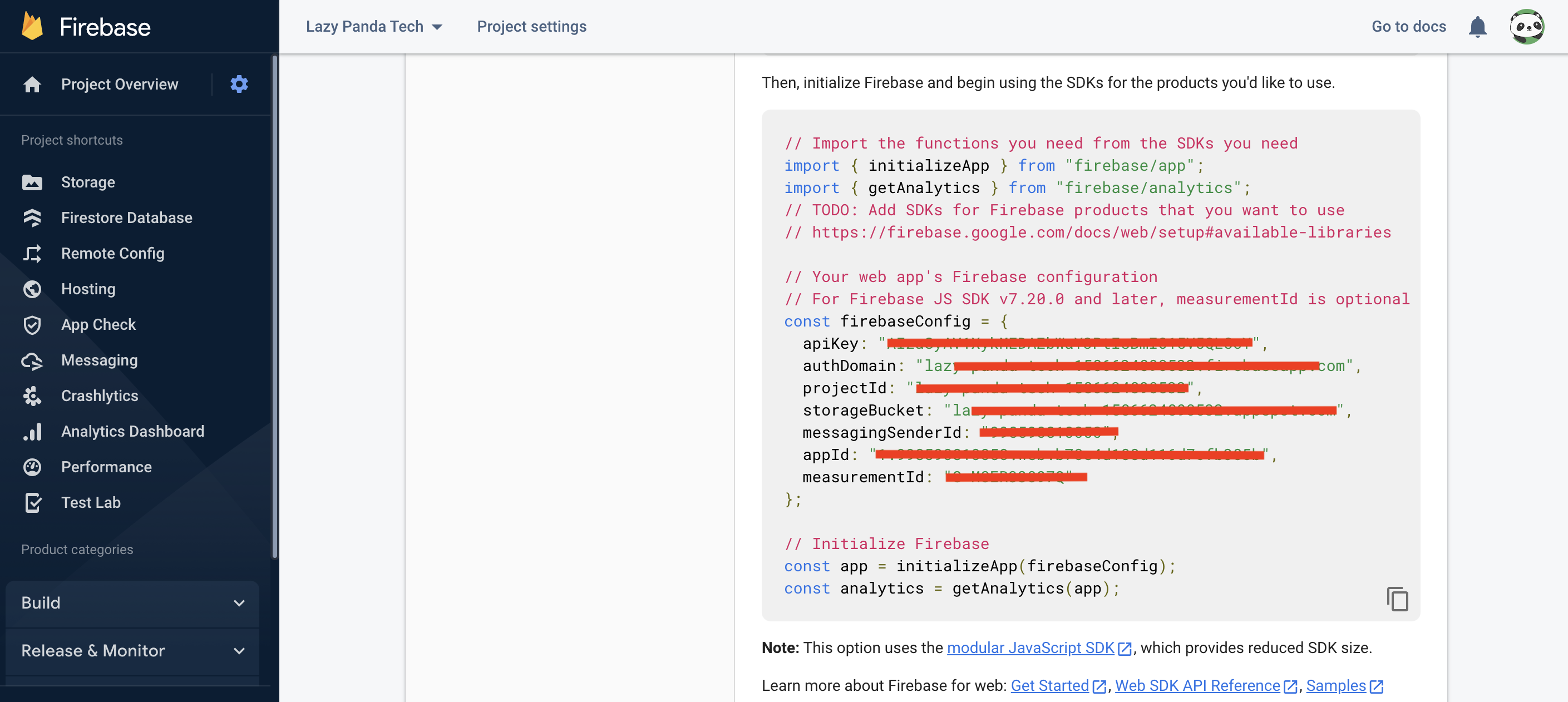
Step 4: Now create a NodeJS & TypeScript application.
I have already prepared a boilerplate Node Js with a TypeScript application. Feel free to check this out.
NodeJS with TypeScript boilerplate code -
Read more.
Step 5: Configure Route path, Multer, and Firebase
In this step, we will write Node Js code and explain how all the component will work together.
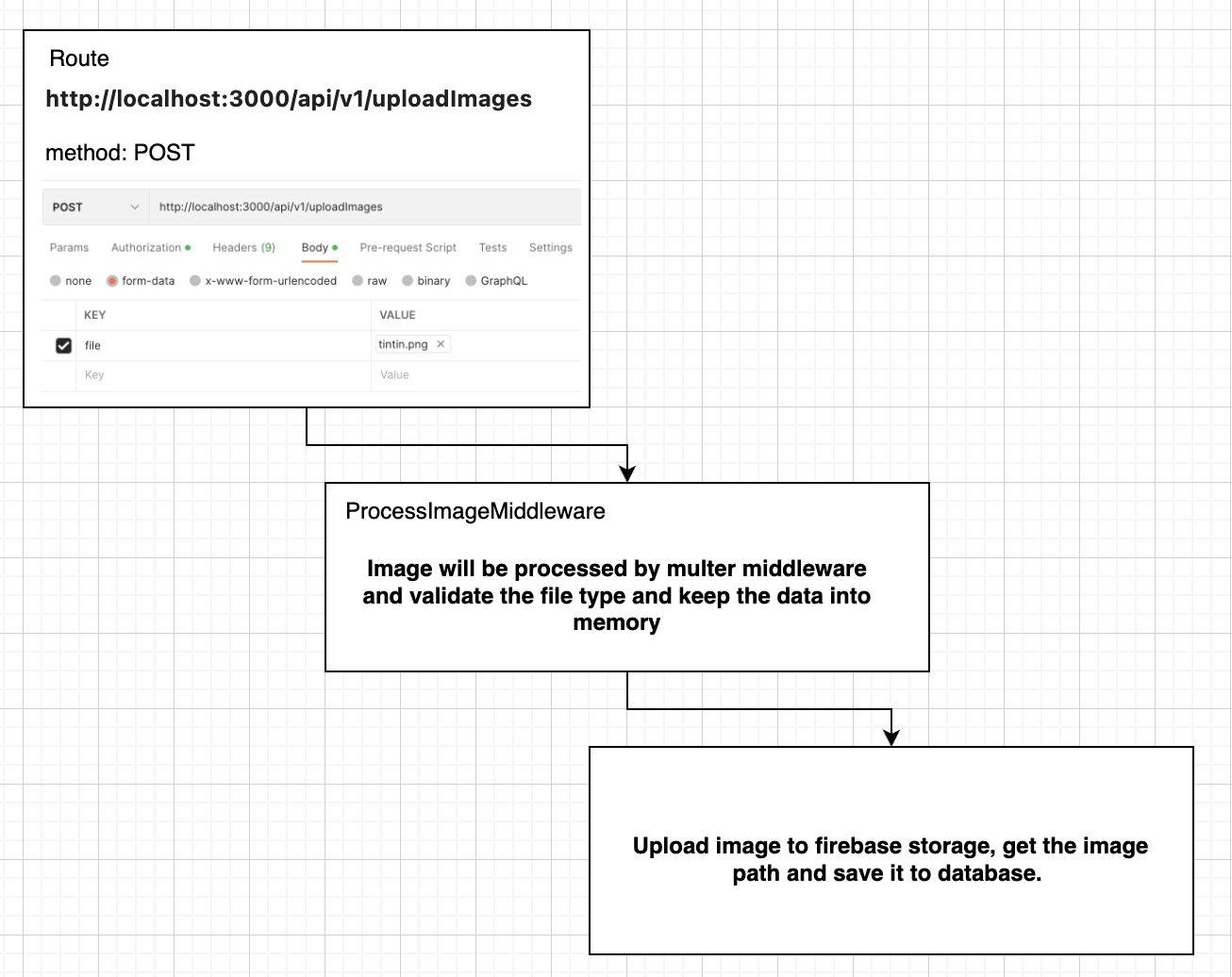
Install the following package.
npm install firebase multer @types/multer
Set up the image process middleware, where we can keep the image filter, memory storage, and limits.
Now, upload the file to Firebase storage, before that if you need you could create a bucket as well.
uploadImageToFirebase(fileDetails: any): Promise<IFileStatus> {
return new Promise<IFileStatus>(async (resolve, reject) => {
const fileStatus: IFileStatus = {
isUploaded: false,
filePath: ''
}
if (!this.isValidRequest) return reject(fileStatus);
// initialize & get reference of firebase storage
const app = initializeApp(this.firebaseConfig);
const storage = getStorage(app);
const storageRef = ref(storage, `lp-blog/${fileDetails.originalname}`);
const metatype = { contentType: fileDetails.mimetype, name: fileDetails.originalname };
await uploadBytes(storageRef, fileDetails.buffer, metatype)
.then(async (snapshot) => {
fileStatus.isUploaded = true;
fileStatus.filePath = `https://storage.googleapis.com/lazy-panda-tech-1586624890532.appspot.com/lp-blog/${fileDetails.originalname}`;
resolve(fileStatus);
})
.catch((error) => {
console.log('Error: ', error);
reject(fileStatus);
});
});
}
Advantages of Using Multer
Using Multer in your Node.js applications simplifies the otherwise complicated process of uploading and saving images directly on your server. Multer is also based on a busboy, a Node.js module for parsing incoming form data, making it very efficient for parsing form data.
Thanks,
-LP
Loading comments...