Create an autocomplete address search using google maps using React
Google place API is one of the best ways to find addresses with suggestive searches. In this article, I will try to demonstrate how the type-ahead search will work using react application.
The goal of this article is as follows -
- Create an account in GCP (Google Cloud Platform)
- Enable google Place API in GCP(Google Cloud Platform)
- Create react application and integrate the search option without displaying any map.
- Restrict users to search in one particular country (Eg. India)
- Display user-selected address.
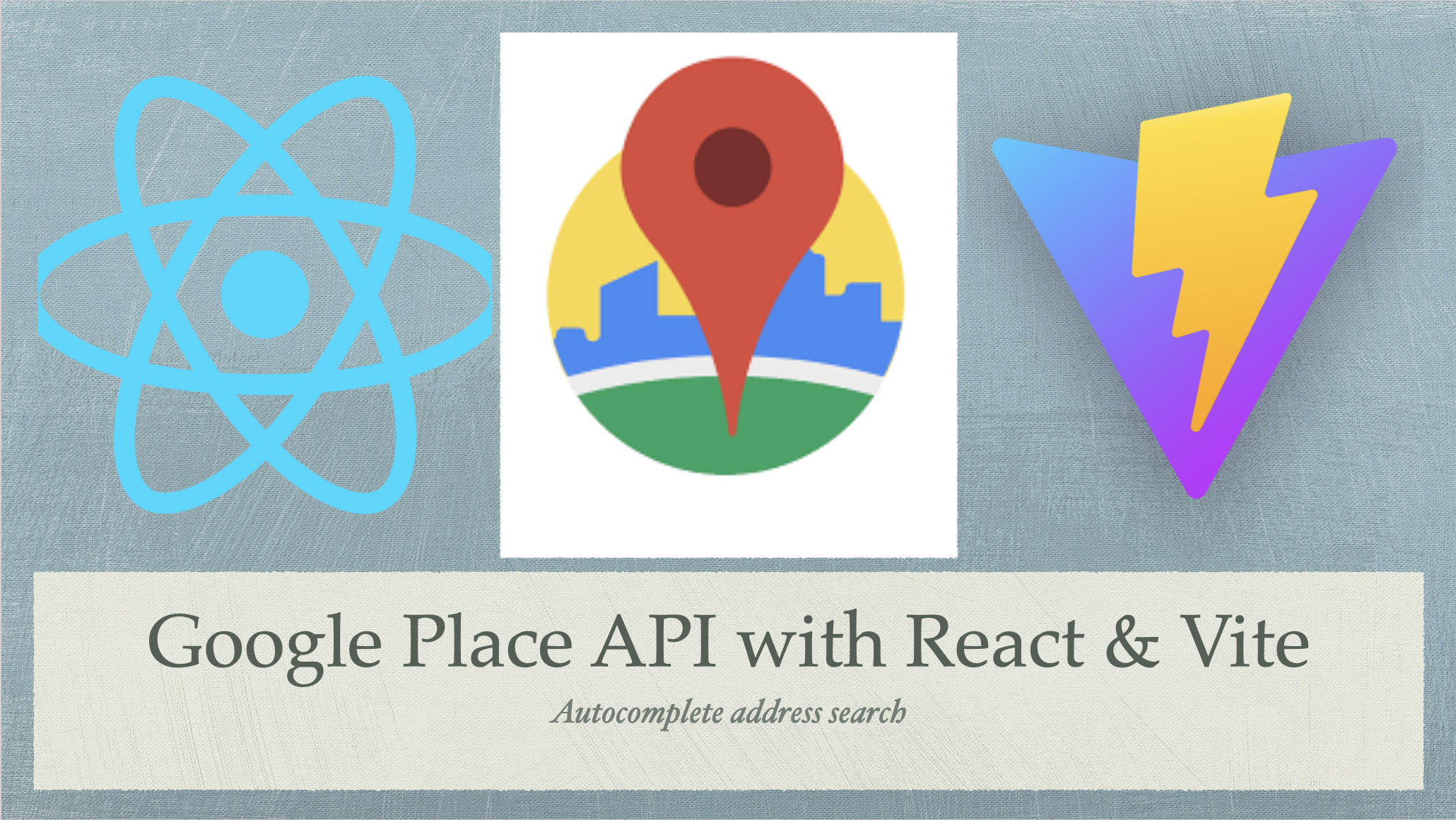
The place autocompletes address search is a very useful Saas product, provided by google and serves data from the same database used by google maps. The service can be used to provide autocomplete capabilities for text-based geographic searches, returning places like businesses, addresses, and points of interest as the user inputs.
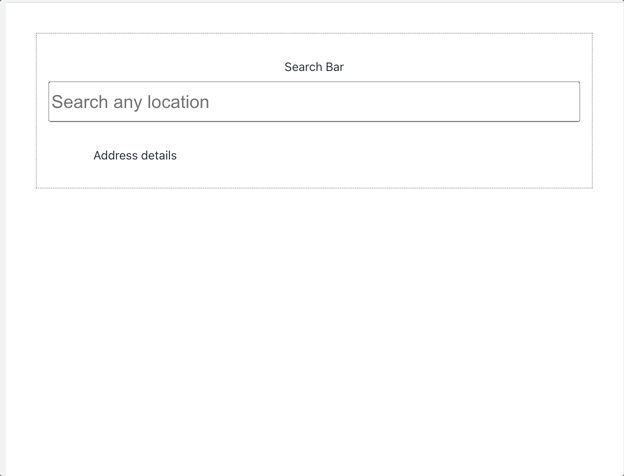
Step 1: Create a User Account in GCP
To leverage Google Place API, we need an API key from Google to leverage the address search feature. Please create an account under Google Developer Console first.
Google Place Autocomplete API is not free (after exceeding the US$200 monthly credit equivalent free usage). To leverage Place API, you need to add the payment information, Google will deduct $1 from your debit or credit card and return back to same account, next time based on user API key usage Google will charge money.
Now coming to pricing, Google has introduced three possible pricing criteria:
- Autocomplete without Places Details – Per Session
- Autocomplete (included with Places Details) – Per Session
- Autocomplete - Per Request
Please visit the map platform site to get more details.
Step 2: Enable Google Place API in GCP(Google Cloud Platform)
You need to enable the place API. The following screenshots might help you to create a Google API key.
Once the API is enabled, you need to create an API key like the one below -
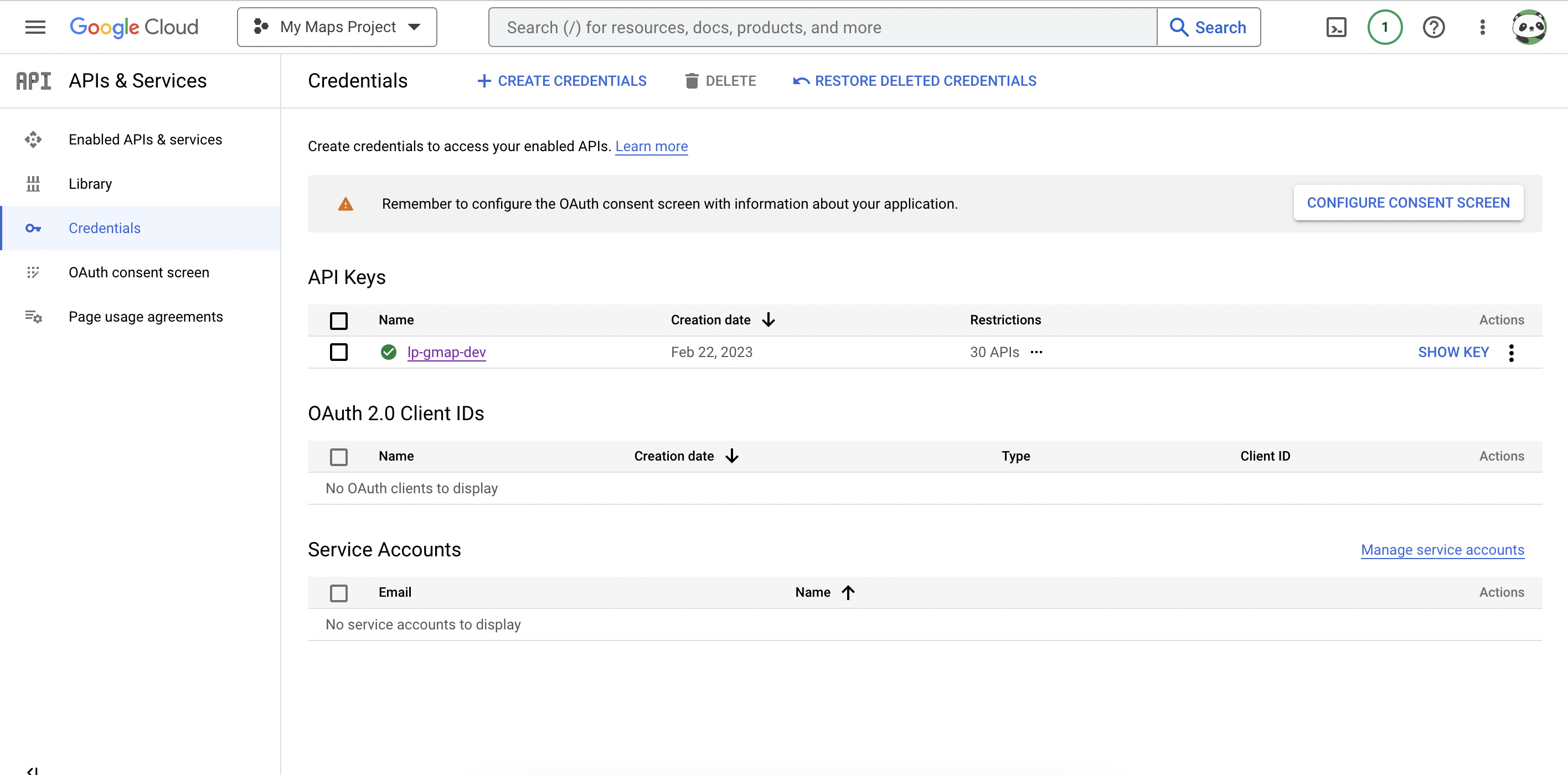
Please copy the API key and add it to your .env file. Also, you can add the restriction to use the API key, so the API will not be misused by unwanted activity.
Step 3: Create react application and integrate the search option without displaying any map.
For this step, I used Vite to generate the react application. After creating the application, I used '@googlemaps/react-wrapper' npm library to configure google Maps. The following diagram will portray to build the application.
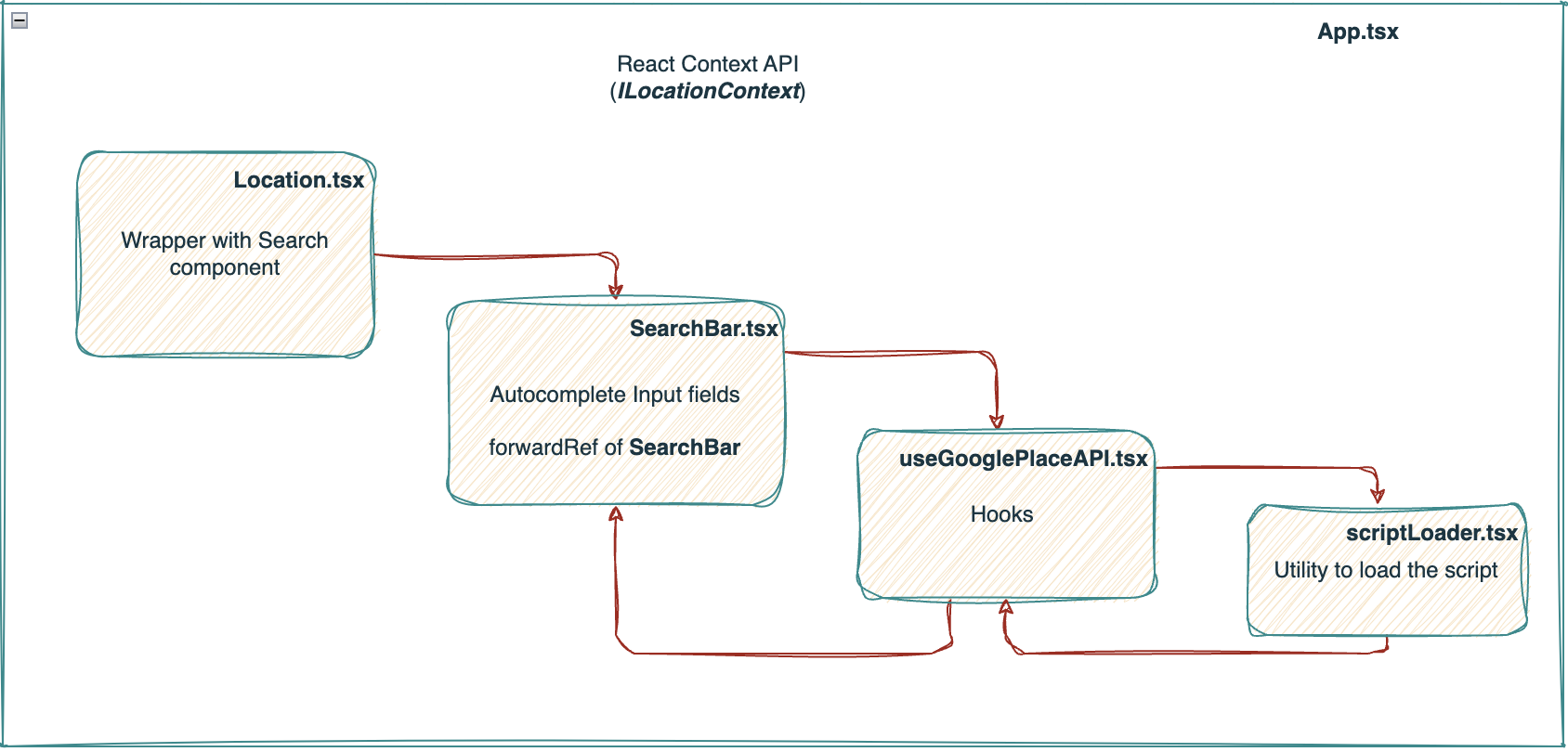
Now, let's create each part and see the example of the code.
Now, the next component is the searchBar, which will be added with forwardRef of same component. like below -
As you can see above, we need to handle the user typings and initialize the google Autocomplete object as well, also we need to add the autocomplete script too. Let's add the custom hooks which will help us to initialize the Autocomplete object.
import { useCallback, useEffect, useRef } from "react";
import { isBrowser, loadGoogleMapScript } from "../utility/loadScript";
const useGoolePlacesAPI = (props: any) => {
const {
ref,
onPlaceSelected,
apiKey,
libraries = 'places',
inputAutocompleteValue = 'new-password',
options: {
types = [],
componentRestrictions,
fields = [
'address_component',
'geometry.location',
'place_id',
'formatted_address',
'name'
],
bounds,
...options
},
gooleMapScriptBaseUrl = 'https://maps.googleapis.com/maps/api/js',
language
} = props;
const inputRef = useRef<HTMLInputElement>(null);
const event = useRef<any>(null);
const autocompleteRef = useRef<any>(null);
const languageQueryParam = language ? `&language=${language}` : '';
const googleMapsScriptUrl = `${gooleMapScriptBaseUrl}?libraries=${libraries}&key=${apiKey}${languageQueryParam}`;
const initializeScript = useCallback(() => {
return loadGoogleMapScript(gooleMapScriptBaseUrl, googleMapsScriptUrl);
}, [googleMapsScriptUrl, gooleMapScriptBaseUrl]);
useEffect(() => {
const config = {
...options,
fields,
types,
bounds
}
if (componentRestrictions) {
config.componentRestrictions = componentRestrictions;
}
if (autocompleteRef.current || !inputRef.current || !isBrowser) return;
if (ref && !ref.current) ref.current = inputRef.current;
const handleAutoComplete = () => {
if (typeof google === 'undefined') return console.error('Google Map has not initialized!');
if (!google.maps.places) return console.error('Google map place API has not been initialized!');
if (!(inputRef.current instanceof HTMLInputElement)) return console.error('Input ref must be an HTMLInputElement');
autocompleteRef.current = new google.maps.places.Autocomplete(inputRef.current, config);
event.current = autocompleteRef.current.addListener('place_changed', () => {
if (onPlaceSelected && autocompleteRef && autocompleteRef.current) {
onPlaceSelected(
autocompleteRef.current.getPlace(),
inputRef.current,
autocompleteRef.current
)
}
})
}
if (apiKey) {
initializeScript().then(() => handleAutoComplete());
}
return () => (event.current ? event.current.remove() : undefined)
}, []);
return {
ref: inputRef,
autocompleteRef
}
}
export default useGoolePlacesAPI;
The above hook will help us to load the Autocomplete object. But we are still missing the script loading, let's add the script.
const isBrowser = typeof window !== 'undefined' && window.document;
const loadGoogleMapScript = (scriptBaseUrl: string, scriptUrl: string) => {
if (!isBrowser) return Promise.resolve();
if (typeof google === 'undefined') return Promise.resolve();
const scriptElement = document.querySelectorAll(`script[src*="${scriptBaseUrl}"]`);
if (scriptElement) {
return new Promise((resolve) => {
if (typeof google !== 'undefined') return resolve(true);
scriptElement[0].addEventListener('load', () => resolve(true));
})
}
const el = document.createElement('script');
el.src = scriptUrl;
document.body.appendChild(el);
return new Promise((resolve) => {
el.addEventListener('load', () => resolve(true));
})
}
export {
isBrowser,
loadGoogleMapScript
}
Now, you are all done. Execute the code and you will see the result on the screen. The complete code can be found in the following GitHub repository too.
Google Map with Autocomplete search, Map and Drag & Drop pin, Example code can be found
here.
Happy Coding!
Loading comments...